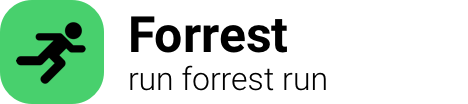
cargo
Cargo is a command-line tool and package manager for Rust, a safe and concurrent programming language. It helps developers to manage creating, building, and sharing Rust projects effectively. Here are some key features and functionalities of Cargo:
-
Dependency Management: Cargo allows you to define and manage dependencies for your Rust projects using the Cargo.toml configuration file. It can automatically fetch and build required dependencies from various sources, such as the official Rust package registry (crates.io) or Git repositories.
-
Build System: Cargo provides a build system that automatically compiles your Rust code into executable binaries or libraries. It handles compilation, linking, and dependency resolution. Additionally, it supports incremental compilation, which speeds up the development process by only recompiling modified code.
-
Testing and Benchmarking: Cargo provides built-in support for running tests and benchmarks for your Rust projects. You can define unit tests, integration tests, and even doctests within your code. Cargo can execute these tests and generate detailed reports.
-
Project Initialization: Cargo allows you to quickly create new Rust projects using the
cargo new
command, which sets up a basic directory structure and initializes a default Cargo.toml file. This makes it easy to start new projects and makes sure they follow best practices. -
Documentation Generation: With the
cargo doc
command, Cargo can generate documentation for your Rust code based on code comments written in Markdown. It automatically generates an HTML version of the documentation that includes modules, functions, types, and their documentation. -
Publish and Share: Cargo makes it simple to publish your Rust packages to the official package registry, crates.io. This allows other developers to easily discover and use your packages in their projects.
Overall, Cargo is an integral part of the Rust ecosystem, providing project management, building, testing, and dependency management capabilities. It streamlines the development process and allows developers to focus on writing safe and efficient Rust code.
List of commands for cargo:
-
cargo-add:tldr:1f337 cargo-add: Add a dependency and enable one or more specific features.$ cargo add ${dependency} --features ${feature_1},${feature_2}try on your machineexplain this command
-
cargo-add:tldr:371d4 cargo-add: Add a development or build dependency.$ cargo add ${dependency} --${select}try on your machineexplain this command
-
cargo-add:tldr:4e610 cargo-add: Add the latest version of a dependency to the current project.$ cargo add ${dependency}try on your machineexplain this command
-
cargo-add:tldr:58df0 cargo-add: Add a local crate as a dependency.$ cargo add --path ${path-to-crate}try on your machineexplain this command
-
cargo-add:tldr:97ac4 cargo-add: Add a dependency with all default features disabled.$ cargo add ${dependency} --no-default-featurestry on your machineexplain this command
-
cargo-add:tldr:ca754 cargo-add: Add a specific version of a dependency.$ cargo add ${dependency}@${version}try on your machineexplain this command
-
cargo-add:tldr:ec1bc cargo-add: Add an optional dependency, which then gets exposed as a feature of the crate.$ cargo add ${dependency} --optionaltry on your machineexplain this command
-
cargo-build:tldr:15d47 cargo-build: Require that `Cargo.lock` is up to date.$ cargo build --lockedtry on your machineexplain this command
-
cargo-build:tldr:348e1 cargo-build: Build a specific package.$ cargo build --package ${package}try on your machineexplain this command
-
cargo-build:tldr:5bdd3 cargo-build: Build only the specified test target.$ cargo build --test ${testname}try on your machineexplain this command
-
cargo-build:tldr:92604 cargo-build: Build artifacts in release mode, with optimizations.$ cargo build --releasetry on your machineexplain this command
-
cargo-build:tldr:9b21d cargo-build: Build the package or packages defined by the `Cargo.toml` manifest file in the local path.$ cargo buildtry on your machineexplain this command
-
cargo-build:tldr:b5f96 cargo-build: Build only the specified binary.$ cargo build --bin ${name}try on your machineexplain this command
-
cargo-build:tldr:e3db4 cargo-build: Build all packages in the workspace.$ cargo build --workspacetry on your machineexplain this command
-
cargo-clippy:tldr:3829f cargo-clippy: Run checks for a package.$ cargo clippy --package ${package}try on your machineexplain this command
-
cargo-clippy:tldr:3915e cargo-clippy: Apply Clippy suggestions automatically.$ cargo clippy --fixtry on your machineexplain this command
-
cargo-clippy:tldr:59dae cargo-clippy: Require that `Cargo.lock` is up to date.$ cargo clippy --lockedtry on your machineexplain this command
-
cargo-clippy:tldr:de155 cargo-clippy: Run checks on all packages in the workspace.$ cargo clippy --workspacetry on your machineexplain this command
-
cargo-clippy:tldr:e19cc cargo-clippy: Run checks over the code in the current directory.$ cargo clippytry on your machineexplain this command
-
cargo-doc:tldr:17bec cargo-doc: Build documentation without accessing the network.$ cargo doc --offlinetry on your machineexplain this command
-
cargo-doc:tldr:548d2 cargo-doc: View a particular package's documentation.$ cargo doc --open --package ${package}try on your machineexplain this command
-
cargo-doc:tldr:6457f cargo-doc: View a particular package's documentation offline.$ cargo doc --open --offline --package ${package}try on your machineexplain this command
-
cargo-doc:tldr:fa25e cargo-doc: Build and view the default package documentation in the browser.$ cargo doc --opentry on your machineexplain this command
-
cargo-rustc:tldr:16bb5 cargo-rustc: Build the package or packages defined by the `Cargo.toml` manifest file in the current working directory.$ cargo rustctry on your machineexplain this command
-
cargo-rustc:tldr:44077 cargo-rustc: Build artifacts in release mode, with optimizations.$ cargo rustc --releasetry on your machineexplain this command
-
cargo-rustc:tldr:7346b cargo-rustc: Build only the specified binary.$ cargo --bin ${name}try on your machineexplain this command
-
cargo-rustc:tldr:b9eb1 cargo-rustc: Build a specific package.$ cargo rustc --package ${package}try on your machineexplain this command
-
cargo-test:tldr:7838d cargo-test: Run tests for a package.$ cargo test --package ${package}try on your machineexplain this command
-
cargo-test:tldr:a72ad cargo-test: Test all packages in the workspace.$ cargo test --workspacetry on your machineexplain this command
-
cargo-test:tldr:a7b55 cargo-test: Test artifacts in release mode, with optimizations.$ cargo test --releasetry on your machineexplain this command
-
cargo-test:tldr:e4fe6 cargo-test: Only run tests containing a specific string in their names.$ cargo test ${testname}try on your machineexplain this command
-
cargo-test:tldr:f1292 cargo-test: Require that `Cargo.lock` is up to date.$ cargo test --lockedtry on your machineexplain this command
-
cargo:tldr:123c7 cargo: Build the rust project in the current directory using the nightly compiler.$ cargo +nightly buildtry on your machineexplain this command
-
cargo:tldr:179b4 cargo: Create a new binary or library Rust project in the specified directory.$ cargo new ${path-to-directory} --${select}try on your machineexplain this command
-
cargo:tldr:19abd cargo: Build using a specific number of threads (default is the number of CPU cores).$ cargo build --jobs ${number_of_threads}try on your machineexplain this command
-
cargo:tldr:282b4 cargo: Install a crate.$ cargo install ${crate_name}try on your machineexplain this command
-
cargo:tldr:8c8c6 cargo: Search for crates.$ cargo search ${search_string}try on your machineexplain this command
-
cargo:tldr:bb316 cargo: List installed crates.$ cargo install --listtry on your machineexplain this command
-
cargo:tldr:fcdf0 cargo: Create a new binary or library Rust project in the current directory.$ cargo init --${select}try on your machineexplain this command